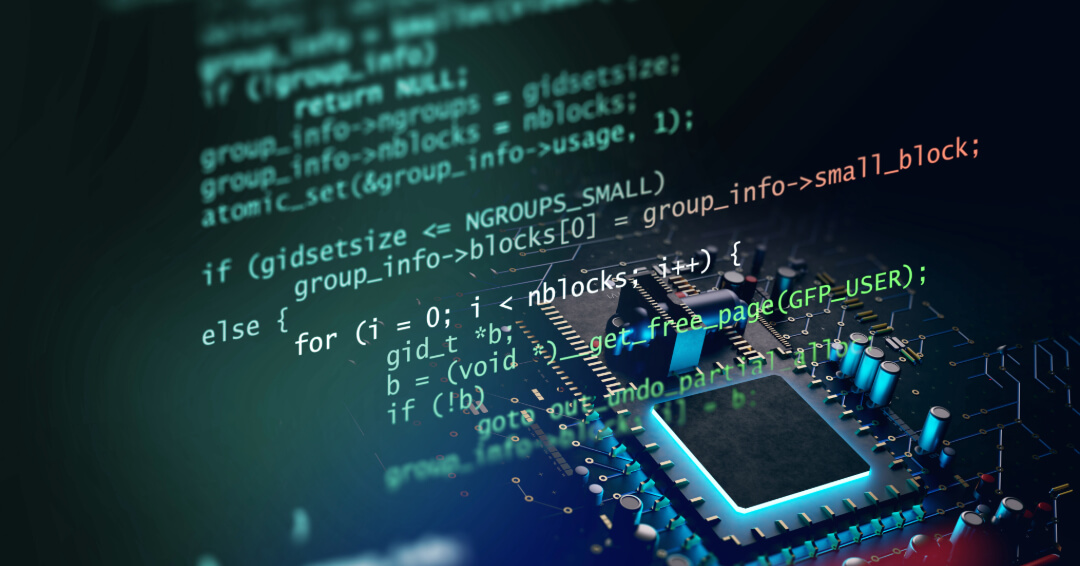
Firmware is a type of hardware-specific software that provides low-level control for electronic devices. Unlike application software, which can be easily changed or updated, firmware is tightly integrated with the hardware and is often found in the ROM or flash memory of the digital device. This software controls the basic functions of the device, such as system startup, power management, and communication with other hardware components.
Embedded devices, which are computational systems designed to perform a specific function, rely heavily on firmware. These devices are used in various technological applications, from microwaves and refrigerators to navigation systems and automobile controllers.
When we talk about abstraction levels in embedded device programming, we refer to the layers at which system developers interact with hardware and software. Each level of abstraction provides a different view of the system, from the most basic hardware to high-level applications, allowing developers to focus on different parts of the system according to their needs. In firmware development, abstraction levels are crucial for simplifying programming and interaction with hardware. These levels enable developers to work more efficiently and create more portable and maintainable code.
1. Hardware Level: This is the lowest level of abstraction. Here, developers work directly with the registers and peripherals of the microcontroller or microprocessor. A deep understanding of the hardware architecture is required, and it is common to use assembly language or C to write code at this level. Developers must manually manage system resources, such as memory and peripherals, which can be complex and error-prone.
2. Hardware Abstraction Level (HAL): HAL is a software layer that provides an interface between the hardware and higher-level software. It allows developers to interact with the hardware without worrying about the specific details of the hardware implementation. HAL uses functions and Application Programming Interfaces (APIs) that facilitate access to peripherals and other hardware functionalities. This not only simplifies development but also enhances code portability, allowing the same firmware to be used on different hardware platforms with minimal modifications.
3. Application Level: At this level, the firmware handles high-level tasks and application logic. The developer works with more abstract interfaces provided by the HAL to interact with the hardware. The focus here is on programming the device's functionality in a general way and the end-user experience. This level includes event handling, user interface management, communication with other devices, and the implementation of the product's main features.
APIs (Application Programming Interfaces) play a fundamental role in embedded firmware development. They provide a standardized way to interact with hardware components and allow developers to write modular and maintainable code. APIs in embedded firmware can be provided by the microcontroller manufacturer or by third-party libraries. Examples of Common APIs: GPIO (General Purpose Input/Output): Allows control of the microcontroller's input and output pins. UART (Universal Asynchronous Receiver-Transmitter): Facilitates serial communication between the microcontroller and other devices. SPI (Serial Peripheral Interface): Used for fast communication between the microcontroller and peripherals such as sensors and memory. I2C (Inter-Integrated Circuit): A communication protocol used to connect multiple devices on a single bus. These APIs greatly simplify development by abstracting specific hardware details and allowing developers to focus on application logic.
Embedded firmware development requires programming languages that offer detailed control over the hardware, high efficiency, and low resource consumption. The most commonly used languages in firmware development are:
Debugging is a critical part of embedded firmware development. Debuggers are tools that allow developers to monitor and control the execution of their code to find and correct errors. Debuggers can be either software or hardware and offer various functionalities:
- Error Detection: They allow identifying and correcting errors in the code, such as stack overflows, memory errors, and logic issues.
- Execution Control: They allow pausing, continuing, and stopping the program execution to analyze its behavior in real-time.
- Memory Inspection: They allow viewing and modifying values in the device's memory, which is essential for detecting errors related to memory management.
- Performance Analysis They help identify bottlenecks and optimize the firmware's performance.
Kinds od Debuggers:
- Software Debuggers Integrated into the Integrated Development Environment (IDE), they allow source-level debugging and are usually sufficient for many projects.
- Hardware Debuggers Like JTAG and SWD, they physically connect to the device and allow for deeper and more precise debugging.
Embedded firmware development follows a lifecycle that can be broken down into several stages:
Especificación de Requisitos:
Identify system needs, define hardware and software specifications, and establish project objectives.
System Design:
Create the hardware and software design, select the microcontrollers and other components, and define the firmware architecture.
Firmware Development:
Write and compile the firmware code using languages such as C or C++, and leverage available APIs and libraries.
Testing and Debugging:
Test the firmware on the actual hardware, use debuggers to identify and correct errors, and ensure the system functions as expected.
Optimization and Validation:
Optimize the firmware's performance, conduct additional tests to ensure the system's stability and reliability, and validate that it meets the specified requirements.
Implementation and Maintenance:
Implement the firmware in the final device, monitor its performance in the field, and perform updates and maintenance as needed.
To ensure quality and efficiency in firmware development, it is important to follow certain best practices:
Code Modularity:
Write modular and reusable code to facilitate the maintenance and expansion of the firmware. Divide the code into separate functions and modules for handling sensors, communication, and application logic.
Documentation:
Maintain good code documentation, including comments and external documentation explaining the firmware's functionality and design. Document the functions and APIs used, as well as the overall code structure.
Continuous Testing:
Conduct continuous testing throughout the development cycle, using unit and integration tests to ensure the correct functionality of the firmware. Implement unit tests for each code module and perform integration tests to verify communication between modules.
Memory Management:
Efficiently manage memory usage, avoiding memory leaks and ensuring that the firmware operates correctly within the hardware limitations. Use memory analysis tools to identify and correct memory management issues.
Handling Errors:
Implement robust error handling that allows detecting and appropriately responding to error conditions in the system. Implement error handling routines to detect and respond to communication failures or faulty sensors.
Security:
Ensure that the firmware is secure and protected against threats by implementing appropriate security measures. Implement encryption for sensitive data communication and ensure that the firmware is not susceptible to code injection attacks.
The following are some practical examples of embedded firmware applications:
Temperature Control System:
* Description: A system that monitors and controls temperature in an industrial plant.
* Components: Sensors, microcontroller, user interface, communication system.
* Process: The firmware reads data from the sensors, adjusts the temperature as needed, and displays the information on a user interface.
* Challenges: Ensure the accuracy of temperature readings, optimize actuator control, and guarantee system reliability.
Home Automation:
* Description: A home automation system that allows controlling lights, appliances, and security systems from a mobile device.
*Components: Microcontroller, communication modules (Wi-Fi, Bluetooth), sensors, and actuators.
* Process: The firmware enables communication between devices and remote control through a mobile application.
* Challenges: Ensure secure and reliable communication, manage multiple devices simultaneously, and optimize energy consumption.
Healt Monitoring System:
* Description: A portable device that monitors vital signs such as heart rate and oxygen saturation.
* Components: Biometric sensors, microcontroller, user interface, communication system.
* Process: The firmware collects and processes data from the sensors, displays the information on a screen, and transmits the data to a mobile application for analysis.
* Challenges: Ensure the accuracy of biometric data, optimize energy consumption for longer battery life, and guarantee the security of health data.
The future of embedded firmware is full of opportunities and challenges. As technology advances, embedded devices are becoming increasingly complex and powerful. Here are some key trends that will shape the future of embedded firmware:
Internet of Things (IoT):
The growing adoption of IoT devices requires firmware that can efficiently handle connectivity, security, and data management.
Inteligencia Artificial (IA):
La integración de IA en dispositivos embebidos permitirá una mayor automatización y capacidades de aprendizaje. Como ejemplo podemos mencionar a sensores inteligentes que pueden aprender y adaptarse a su entorno para mejorar la precisión y la eficiencia.
Security:
Security will be an increasing priority as embedded devices are more exposed to threats and attacks. For example, the implementation of robust security measures such as encryption and multi-factor authentication can be mentioned.
Power Eficiency:
The demand for portable and low-power devices will drive the development of more energy-efficient firmware. For example, portable medical devices that can operate for long periods on small batteries can be mentioned.
Over-The-Air Firmware Update:
The ability to update firmware remotely will be essential for maintaining the functionality and security of embedded devices. For example, connected cars that receive software and firmware updates to enhance their functions and security can be mentioned.
Embedded firmware is essential for the operation of modern devices, providing the necessary control for these devices to function correctly. Understanding abstraction levels, APIs, programming languages, and debugging tools is crucial for any developer in this field. Additionally, following good development practices and keeping up with emerging trends will enable developers to create more efficient, secure, and functional firmware.